如何在 Python 中創建提示和拆分計算器
已發表: 2023-01-06讓我們學習如何在 Python 中創建 Tip and Split 計算器。
這是一個很棒的個人項目,可以用來練習您的 Python 技能。 此外,本教程將教您如何以兩種方式創建應用程序,第一種是作為命令行工具,第二種是作為 GUI 工具。
預習
我們將以兩種方式構建應用程序。 首先,我們將構建一個簡單的 Python shell 腳本,提示用戶輸入並寫入輸出。
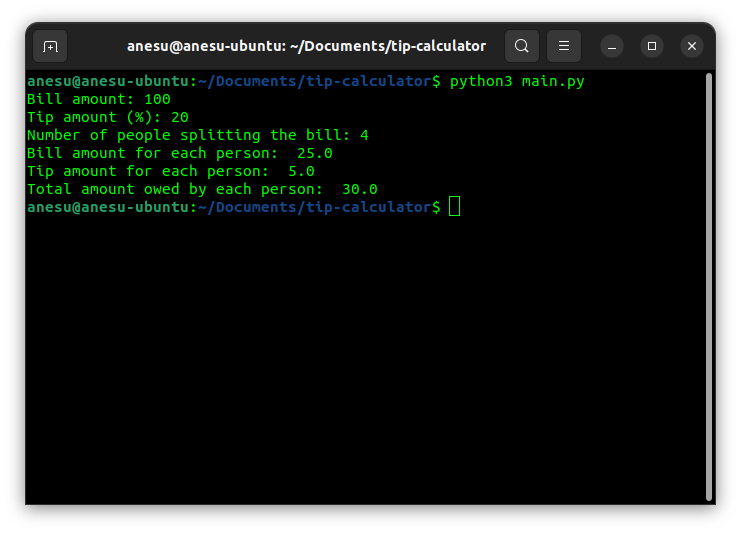
其次,我們將使用 Tkinter 為程序提供圖形用戶界面。
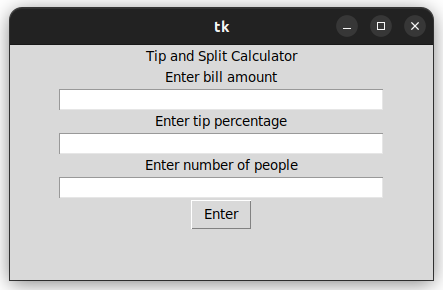
程序規範
該程序接收三個輸入:
- 賬單金額
- 小費百分比
- 分擔賬單的人數
使用這些輸入,程序將計算以下輸出:
- 每個人對賬單的貢獻
- 每個人對小費的貢獻
- 每個人的總貢獻
算法
為此,Tip and Split 計算器將遵循下面概述的非常簡單的算法:
- 接收輸入
: bill_amount
、tip_percentage
、number_of_people
- 通過乘以
bill_amount
*tip_percentage / 100
計算小費amount
- 將
bill_amount
除以number_of_people
得到每個人對賬單的貢獻。 - 將
tip_amount
除以number_of_people
得到每個人對小費的貢獻。 - 最後,將對賬單的貢獻和小費相加以獲得應付總額。
先決條件
要學習本教程,您應該知道並理解 Python 編程語言。 對於本教程,需要了解基本概念,包括函數和類。
此外,您的系統中應該安裝了 Python。 如果不是,請轉到 Python 網站並下載它。 或者,Geekflare 有一個在線 Python 編譯器,您可以在瀏覽器中運行您的 Python 代碼,而無需任何環境設置。
使用命令行界面構建計算器
創建項目文件夾
首先,導航到系統中的一個空文件夾。 在我的例子中,我使用的是 Ubuntu 22.04,所以要創建一個文件夾並使用終端導航到它; 我需要輸入以下命令:
mkdir tip-calculator && cd tip-calculator
創建 Python 文件
接下來,創建我們將在其中編寫 Python 腳本的腳本文件。 就我而言,我將使用touch
命令來執行此操作:
touch main.py
使用您喜歡的代碼編輯器打開腳本文件
要開始將代碼寫入腳本,請使用您喜歡的代碼編輯器打開文件。 我將使用nano
,它是一個基於終端的文本編輯器。
nano main.py
接收輸入
完成後,我們可以將以下代碼行添加到文件頂部:
# Receiving input for bill amount as a floating point number bill_amount = float(input("Bill amount: ")) # Receiving input for the tip percentage as a floating point number tip_percentage = float(input("Tip percentage: ")) # Receiving the input for the number of people as an integer number_of_people = int(input("Number of people: "))
基本上,它接收輸入並將每個輸入的數據類型從字符串轉換為最合適的類型。
計算小費金額
接下來,我們通過將小費百分比乘以賬單金額來計算小費金額。
tip_amount = bill_amount * tip_percentage / 100
劃分賬單和小費以獲得每個人對兩人的貢獻
# Calculating each person's bill contribution bill_contribution = bill_amount / number_of_people # Calculating each person's tip contribution tip_contribution = tip_amount / number_of_people
計算總貢獻
接下來,添加個人貢獻以確定每個人的總貢獻。
total_contribution = bill_contribution + tip_contribution
顯示結果
最後將結果輸出給用戶。
# Displayinnng the results print("Bill contribution per person: ", bill_contribution) print("Tip contribution per person: ", tip_contribution) print("Total contribution per person: ", total_contribution)
測試 Tip and Split 計算器
最後,您的腳本文件應如下所示:
# Receiving input for bill amount as a floating point number bill_amount = float(input("Bill amount: ")) # Receiving input for the tip percentage as a floating point number tip_percentage = float(input("Tip percentage: ")) # Receiving the input for the number of people as an integer number_of_people = int(input("Number of people: ")) tip_amount = bill_amount * tip_percentage / 100 # Calculating each person's bill contribution bill_contribution = bill_amount / number_of_people # Calculating each person's tip contribution tip_contribution = tip_amount / number_of_people total_contribution = bill_contribution + tip_contribution # Displaying the results print("Bill contribution per person: ", bill_contribution) print("Tip contribution per person: ", tip_contribution) print("Total contribution per person: ", total_contribution)
此時,請隨時使用以下命令測試運行您的應用程序:

python3 main.py
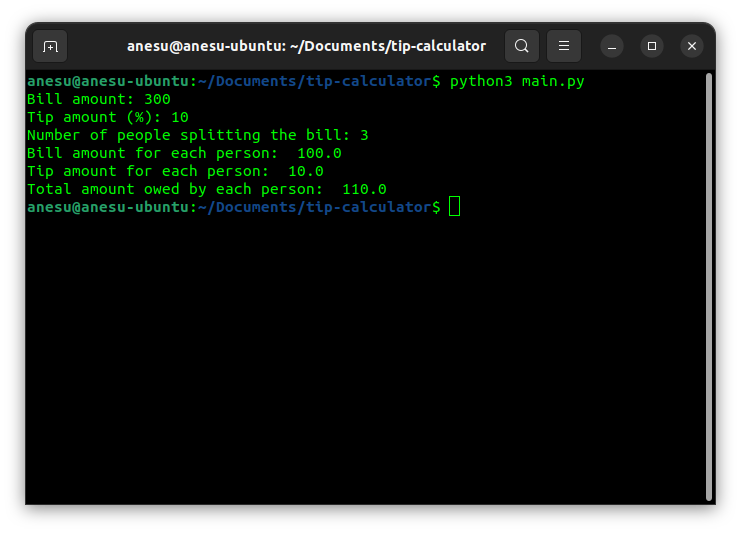
使用 GUI 構建 Tip and Split 計算器
在本教程的下一部分中,我們將實現相同的應用程序,但使用圖形用戶界面。 要構建 GUI,我們將使用一個名為 Tkinter 的包。
配置
Tkinter 是內置於 Python 標準庫中的一個包。 這意味著它是在您安裝 Python 時默認安裝的。
然而,在默認安裝了 Python 的 Linux 機器上,TKinter 並沒有預裝以節省空間。 因此,您需要使用以下命令手動安裝它:
sudo apt-get install python3-tk
創建項目文件
首先,創建一個用於存儲 Python 腳本的文件。 創建文件後,使用您喜歡的文本編輯器打開它。
touch gui.py
導入 Tkinter
接下來,通過將以下行添加到文件頂部來導入 Tkinter 包。
import tkinter from tk
創建用戶界面
然後,我們可以開始創建用戶界面。
# Creating the window window = tk.Tk() # Creating the Window title tk.Label(text="Tip and Split Calculator").pack() # Create an input field tk.Label(text="Enter bill amount").pack() ent_bill = tk.Entry(width=40) ent_bill.pack() # Create and entry for the tip percentage tk.Label(text="Enter tip percentage").pack() ent_tip = tk.Entry(width=40) ent_tip.pack() # Create an entry for the number of people tk.Label(text="Enter the number of people").pack() ent_people = tk.Entry(width=40) ent_people.pack() # Create the Enter button btn_enter = tk.Button(text="Enter")
上面的代碼創建了一個包含所有用戶界面小部件的窗口。 此外,它還創建了一個將用作窗口標題的標籤。
接下來,它為三個輸入創建了標籤和輸入字段: bill_amount
、 tip_percentage
和number_of_people
。 最後,它創建了一個按鈕,用戶可以單擊該按鈕來運行計算。
創建一個函數來計算輸出
在此之後,我們可以創建一個函數來處理 Enter 按鈕的點擊。 此函數將獲取輸入字段的值,並使用它們使用前面提到的算法計算輸出。 最後,它將創建一個標籤來顯示輸出並更新窗口。
def handle_click(event): # Collecting the inputs from the entry fields using the get method # Also type casting the inputs from the default string data type bill_amount = float(ent_bill.get()) tip_percentage = float(ent_tip.get()) number_of_people = int(ent_people.get()) # Calcuating the amount to be paid as a tip tip_amount = bill_amount * tip_percentage / 100 # Calculating the bill contribution of each person at the table bill_contribution = bill_amount / number_of_people # Calculating the tip contribution of each person at the table tip_contribution = tip_amount / number_of_people # Calculating the total contribution of each person total_contribution = bill_contribution + tip_contribution # Creating the output string output = f'Bill per person: {bill_contribution} \n Tip per person: {tip_contribution} \n Total per person: {total_contribution}' # Creating a label for the output text tk.Label(text=output).pack() # Updating the window to reflect the UI changes window.update()
上面函數中的代碼已經通過解釋每個主要步驟的註釋進行了解釋。
將事件處理程序附加到按鈕
接下來,我們將事件處理程序綁定到按鈕單擊事件。 Tkinter 中的按鈕點擊事件由字符串“ <Button-1>
”表示。 要將事件綁定到事件處理程序,我們使用按鈕的綁定方法。 在函數定義下方添加這行代碼:
btn_enter.bind('<Button-1>', handle_click) btn_enter.pack()
最後,為了保持窗口運行,我們調用window
對象的mainloop
方法。
window.mainloop()
我們完成了!
測試 Tip and Split 計算器
您可以使用以下命令運行該應用程序:
python3 gui.py
這應該打開如下窗口:
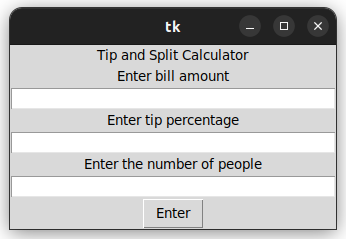
您可以使用示例輸入運行計算器:
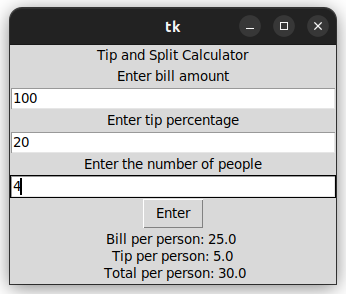
最後的話
在本教程中,我們以兩種方式創建了小費和拆分計算器。 第一個使用基於終端的 CLI 工具。 第二個是使用 Python 的 Tkinter 的 GUI 工具。 本教程展示瞭如何構建一個簡單的 Python 項目。 如果您需要溫習或提高您的 Python 技能,這裡有一個 Datacamp 課程。
接下來,您可以查看如何在 Python 中創建隨機密碼生成器。